API Source Code to Website Help Pages in ASP.NET Web API
Alex Sparkman (@alexpsparkman)
may 2nd, 2014
Recently, I was working on a team project with a number of independent components each with their own data, logic, and presentation layer. I was assigned the task of creating an API for capturing large amounts of real-time data. Since other developers needed to use it, the API had to be documented.
Technical writing is probably one of the most difficult things to do. The intended audience most likely does not want to read it. It needs to have just enough detail, but it needs to be short. And even if it does meet all those requirements, people still may not read it.
The Problem
C# has comments, and then it has documentation comments. The documentation comments may be extracted into an XML file using project settings (howto). The XML file can then be parsed to produce documentation.
Neither C# or Visual Studio provide a method to parse the XML file into a human-readable format. This is where a little research was needed. I found a couple of solutions.
The Search
The first solution was to use an open source project to parse them into a javadoc / MSDN-like format. A stackoverflow post led me to a number of potential packages. The problem was that they revealed too much. It also required a decent amount of knowledge regarding how ASP.NET MVC works. Not everyone on the project knew C#, or ASP.NET MVC for that matter.
The second solution was to use the NuGet package ASP.NET Web API 2 Help Page. This didn’t do what I needed it to. This sample explains why:
/// <summary>
/// Represents a product.
/// </summary>
public class Product
{
/// <summary>
/// The unique identifier for the product.
/// </summary>
public int Id { get; set; }
/// <summary>
/// The name of the product. This does not have to be null. This
/// should be prefixed with its one letter type identifier. This
/// needs to be less than 200 characters in length.
/// </summary>
public string Name { get; set; }
}
Product Code
// POST api/Products
/// <summary>
/// A new product to add.
/// </summary>
/// <param name="product">The product to add</param>
/// <returns>Status code 201 if it was created, along with its ID, and the created property values.</returns>
[ResponseType(typeof(Product))]
public async Task<IHttpActionResult> PostProduct(Product product)
{
if (!ModelState.IsValid)
{
return BadRequest(ModelState);
}
db.Products.Add(product);
await db.SaveChangesAsync();
return CreatedAtRoute("DefaultApi", new { id = product.Id }, product);
}
Function for API Call
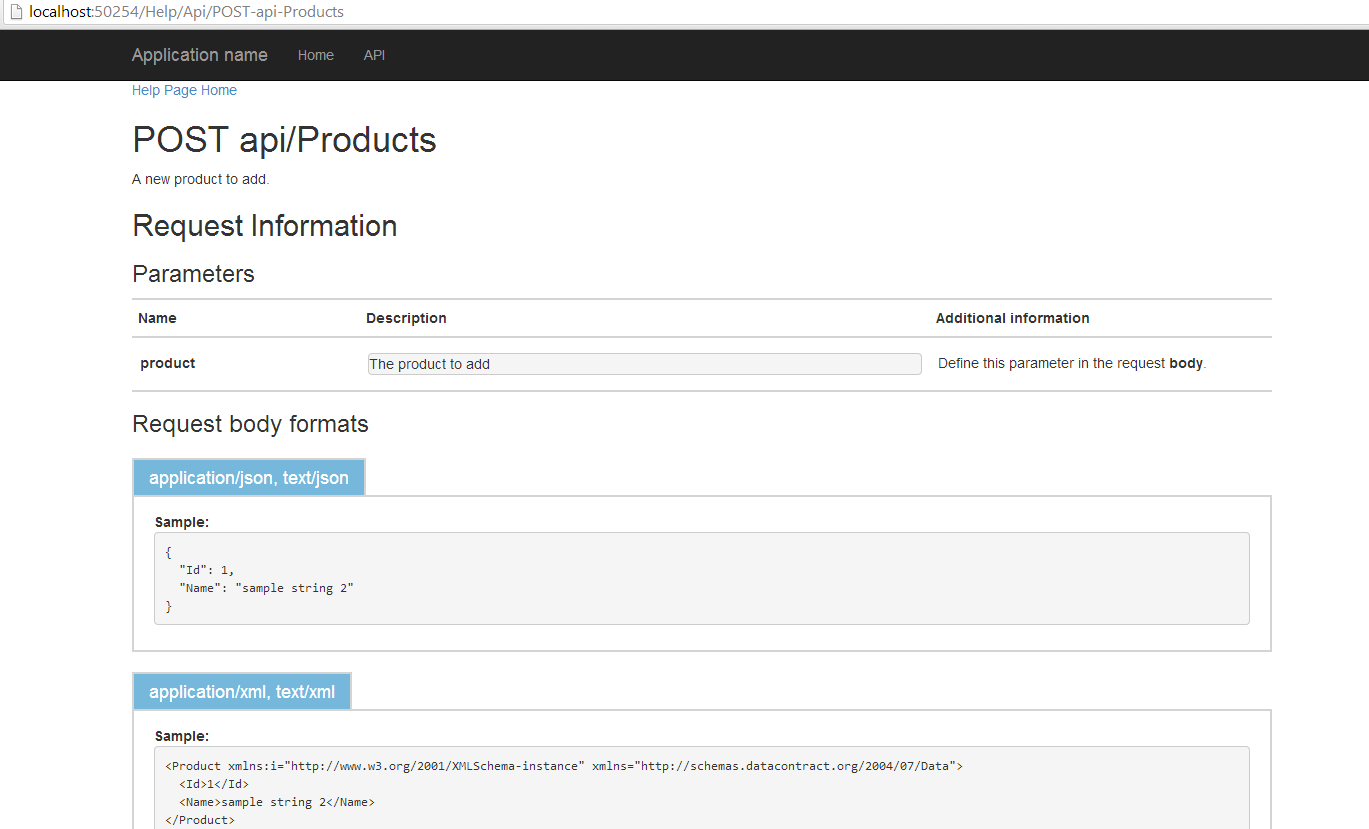
Generated Documentation
The problem was that the generated documentation showed the description for parameters from the PostProduct method, and not the documentation comments from the plain old class. In the sample, the developer using the call would be completely unaware that the Name property needed to be less than 200 characters, and prefixed with its one letter product type identifier.
I did some more research, which brought me to my third attempted solution. The next version, 2.1, did the following:
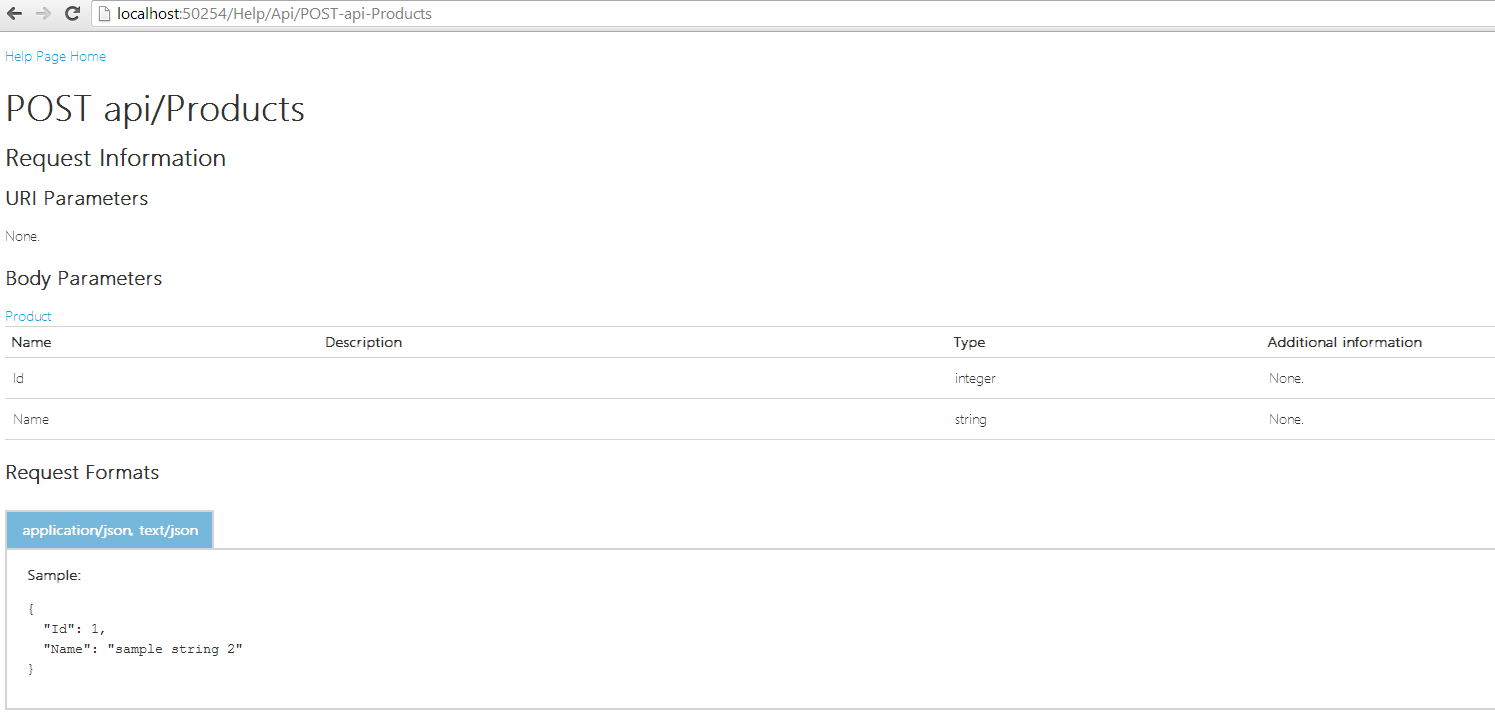
Generated Documentation 2.1
The property comments were not being included, but why? The data class was in a different project. When the XML files are generated, they are generated per project. The help page package can not handle more than one documentation source.
Almost all of the package code is added through source files included in the project. This allowed me to add a second XML file to the documentation input. When it didn’t find the documentation from the first file, it would search the second file.
I changed five lines of the package’s code. The following shows the end result:
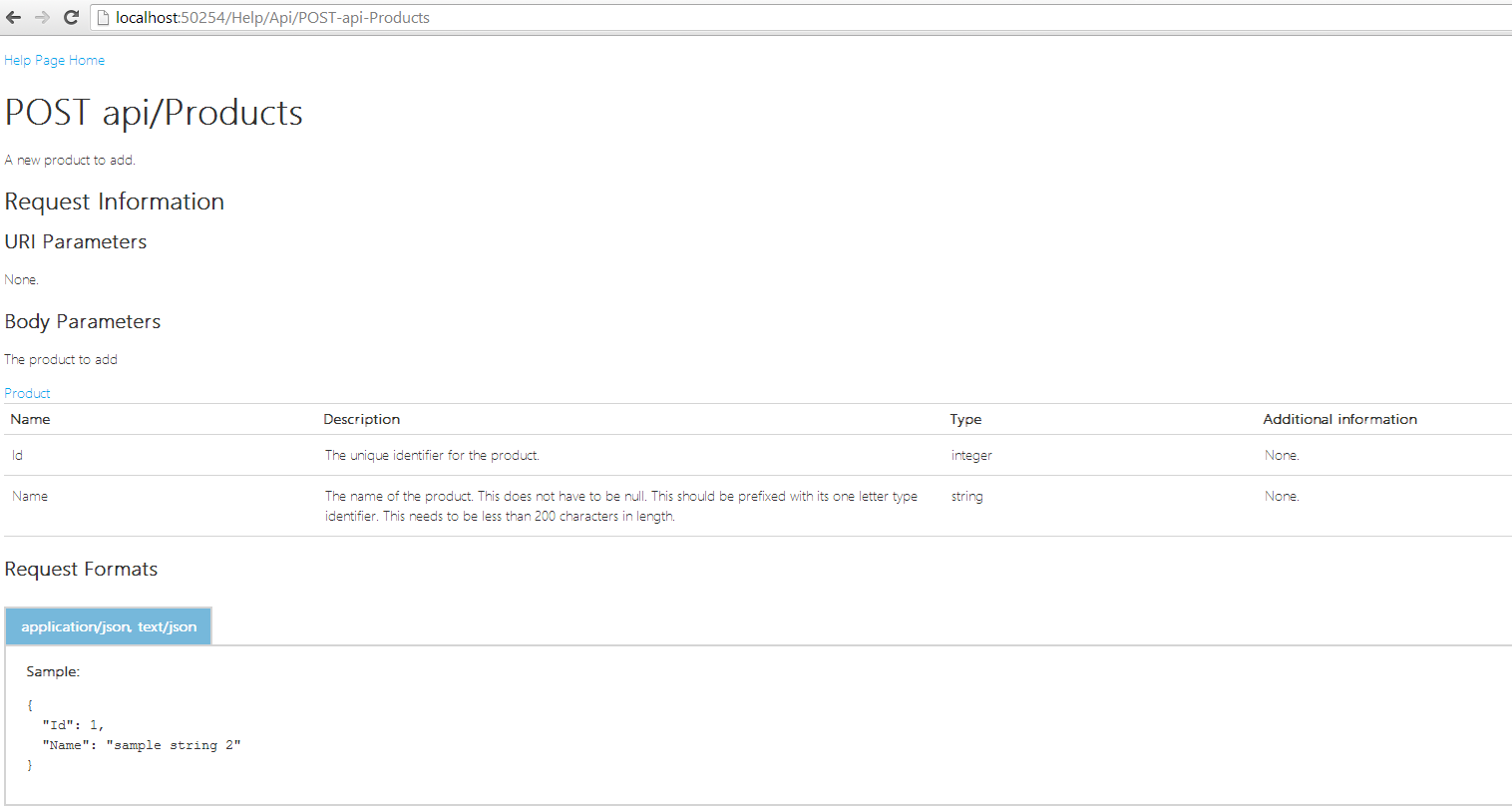
The Result
- If a developer has access to the API, they also have access to the most recent documentation.
- I no longer have to worry about updating separate documents.
- Intelli-sense has useful comments to include when writing new API code that uses the old code.
- When modifying the existing API code, future programmers will have more information from the inline comments.
Tags: technology csharp documentation